Dev Log: Skeletons
This past week I've been chipping away at some general changes to Otter as well as doing some quick experiments with some things that I eventually want to include in my bigger game project.
The most recent of these experiments is setting up a general purposed skeleton system. The main idea is that I want to be able to make up enemies and other objects with a bunch of individual pieces that move around and animate. Normally I could say just using something like Spine or Spriter is good enough for this, but for this I wanted something of my own creation.
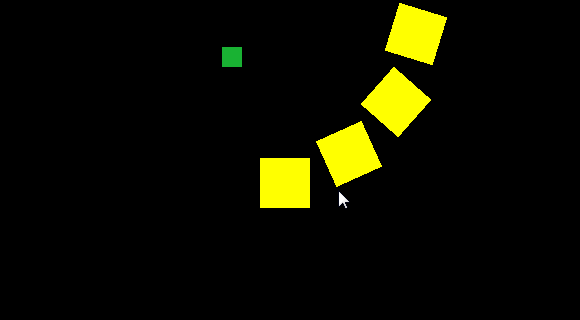
The idea is that there is a Skeleton Entity in Otter that contains bones that all move, rotate, and scale based on their parent. Typical skeleton bone behavior. The difference is that each bone can hold an Entity on it. Each individual Entity on the bone can then have its own logic, so for example the first thing I want to have is the ability to destroy individual pieces of the skeleton. I can do that by having the Entities on each bone have logic for taking damage and being destroyed.
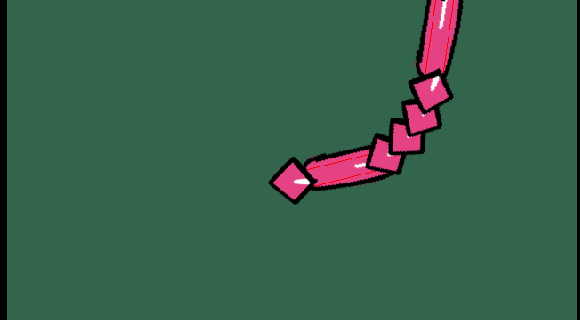
So far I have one early iteration of this working but I'm realizing that the math that programs like Spine and Spriter do might be slightly different, and I'm trying to figure out exactly how they approach their transformations. It's a little difficult to find any articles or tutorials on how to code this stuff up yourself, as most people assume that using Spine or Spriter is what you want to do. I could potentially go back to using Spine and try to figure out how to wrangle its data format into loading a Skeleton into my custom format, but sometimes just making something yourself from scratch ends up being more straight forward!
The most recent of these experiments is setting up a general purposed skeleton system. The main idea is that I want to be able to make up enemies and other objects with a bunch of individual pieces that move around and animate. Normally I could say just using something like Spine or Spriter is good enough for this, but for this I wanted something of my own creation.
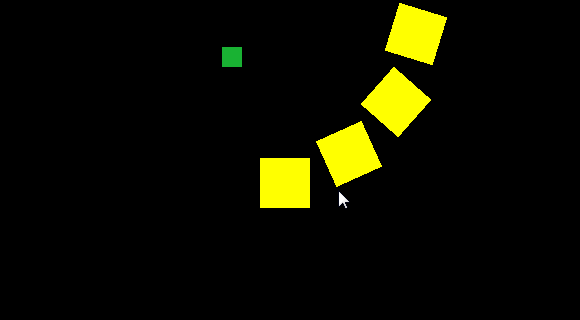
The idea is that there is a Skeleton Entity in Otter that contains bones that all move, rotate, and scale based on their parent. Typical skeleton bone behavior. The difference is that each bone can hold an Entity on it. Each individual Entity on the bone can then have its own logic, so for example the first thing I want to have is the ability to destroy individual pieces of the skeleton. I can do that by having the Entities on each bone have logic for taking damage and being destroyed.
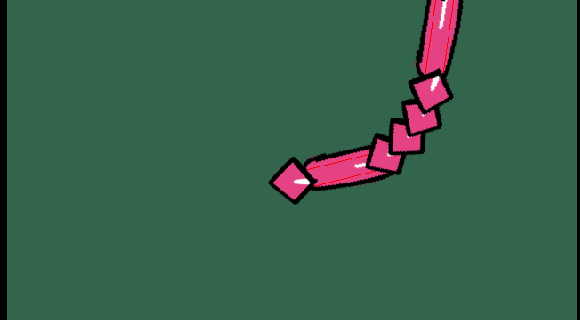
So far I have one early iteration of this working but I'm realizing that the math that programs like Spine and Spriter do might be slightly different, and I'm trying to figure out exactly how they approach their transformations. It's a little difficult to find any articles or tutorials on how to code this stuff up yourself, as most people assume that using Spine or Spriter is what you want to do. I could potentially go back to using Spine and try to figure out how to wrangle its data format into loading a Skeleton into my custom format, but sometimes just making something yourself from scratch ends up being more straight forward!
No Comments